C++ and Nix
Template Project in C++ using Nix
Taken from this blog post.
Creating a C++ project
Create a sample C++ project with the following dependencies
- C++ compiler, of course. That might be GCC or Clang.
- Boost
- Poco
The program does nothing more than printing what compiler it was built with and which versions of boost and poco it is linked against.
Code
|
|
nix-shell
We can either install a C++ compiler by running nix-env with the appropriate arguments, or just run a shell that exposes a C++ compiler in its PATH environment. Let us not clutter the system’s PATH environment with compilers from the beginning, because often people would use different compilers for each project anyway.
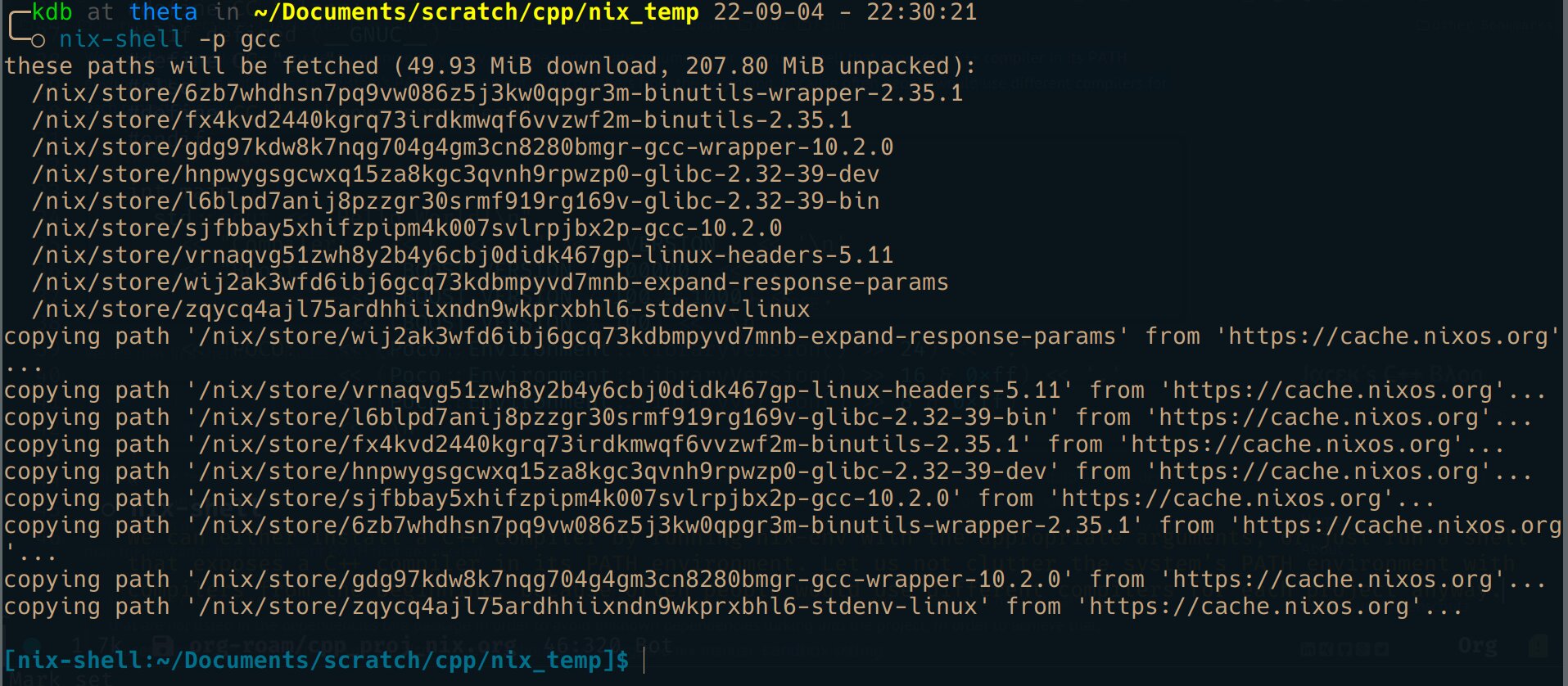
Path
The path list is full of paths that begin with nix/store…. Each of them could be considered what one would call a package on typical Linux distributions. We can easily install multiple compilers with different versions, or even the same version with different sets of patches applied, next to each other in /nix/store and not have any of them collide during a project’s build, because nix does simply only map the packages into the current PATH that are needed.
Run
To compile and run:
nix-shell -p gcc poco boost
c++ -o printEnv printEnv.cpp -lPocoFoundation -lboost_system
- ./printEnv
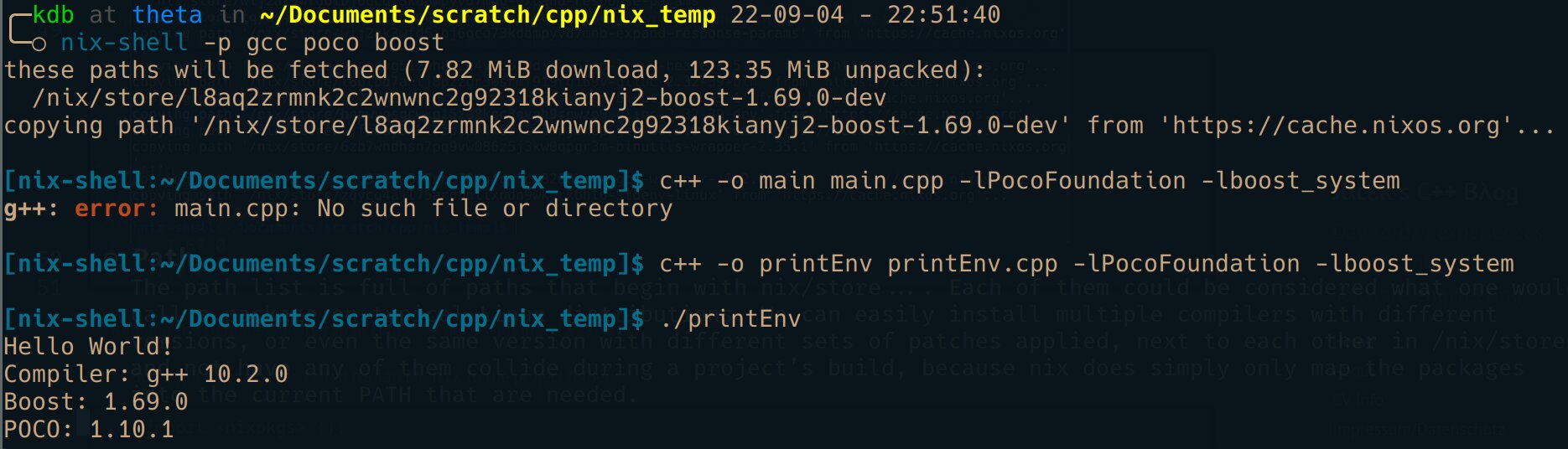
default.nix
|
|
Then simply run nix-build
.